About Lesson
CSS Media Queries:
CSS Media Queries are a feature in CSS that allows you to apply styles based on the characteristics of the device or the media displaying the page. With media queries, you can create responsive designs that adapt to different screen sizes, resolutions, and other device-specific features.
Syntax:
Media queries are written using the @media rule, followed by a media type and/or media feature. The general syntax is:
CSS
@media media-type and (media-feature) {
/* CSS rules to apply when the media type and feature match */
}
Here, media-type could be screen, print, speech, etc., and media-feature could be characteristics like max-width, min-width, orientation, etc.
Example 1:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css"/>
<title>Media Queries Example</title>
</head>
<body>
<h1>Responsive Design Example</h1>
<p>This is a simple example of CSS media queries.</p>
</body>
</html>
CSS
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 20px;
}
/* Default styles for larger screens */
body {
background-color: lightblue;
}
h1 {
color: darkblue;
}
/* Media query for screens with a maximum width of 600px */
@media screen and (max-width: 600px) {
body {
background-color: lightcoral;
}
h1 {
color: darkred;
}
}
Output:
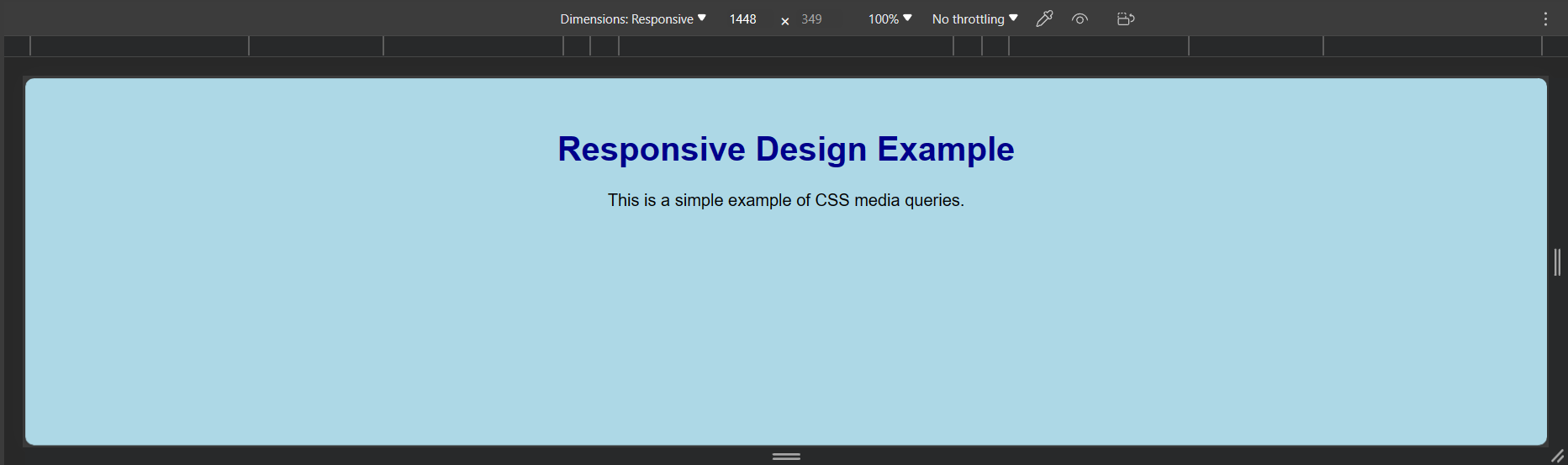
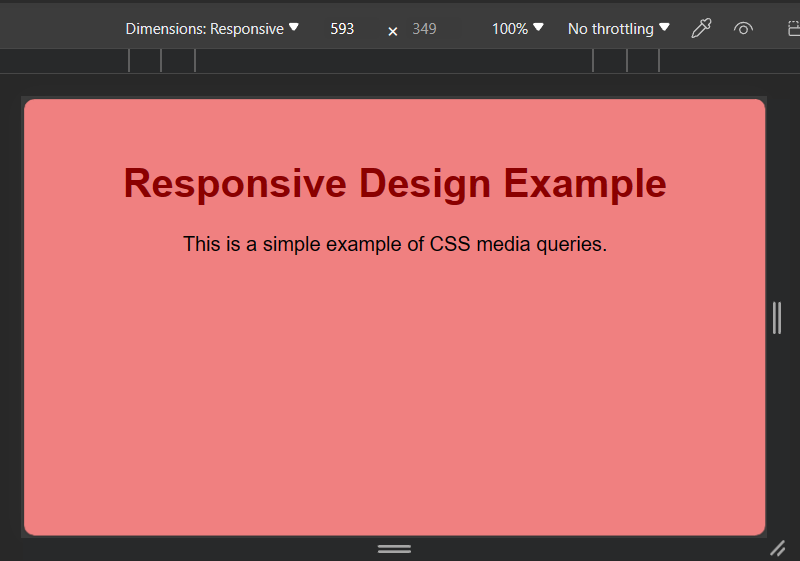
Example 2:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css"/>
<title>Media Query Example 2</title>
</head>
<body>
<div class="container">
<div id="navleft">
<ul id="nav">
<li class="navitem">Menu-item 1</li>
<li class="navitem">Menu-item 2</li>
<li class="navitem">Menu-item 3</li>
<li class="navitem">Menu-item 4</li>
<li class="navitem">Menu-item 5</li>
</ul>
</div>
<div id="main">
<h1>MEDIA QUERY</h1>
<p>When screen size is 480px and more than 480px then content of .main id float left.</p>
</div>
</body>
</html>
CSS
#navleft {
float: none;
width: auto;
background-color: black;
}
.navitem {
border: 1px solid black;
color: white;
border-radius: 4px;
list-style-type: none;
margin: 4px;
padding: 2px;
}
@media screen and (min-width: 480px) {
#navleft {
float: left;
padding: 30px;
}
}
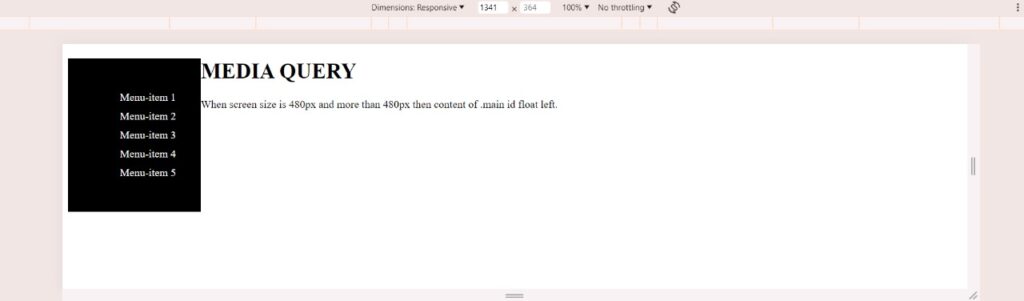
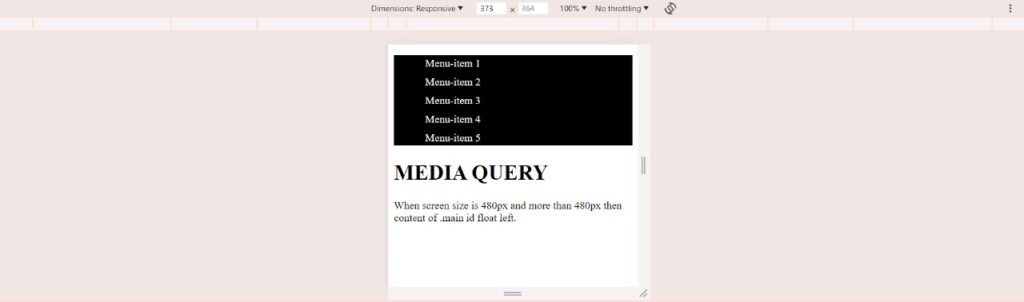