What is PHP Date and Time :
In PHP, handling date and time is essential for various web applications, ranging from displaying current dates to managing time-sensitive operations and scheduling tasks. PHP offers robust built-in functions and classes for working with dates and times.
Current Date and Time:
To get the current date and time, you can use the date() function:
<?php
$currentDate = date('Y-m-d'); // Output: 2024-01-29
$currentTime = date('H:i:s'); // Output: 14:30:00
?>
Formatting Dates:
The date() function allows you to format dates and times according to a specified format string. Common format characters include:
- Y: Four-digit year (e.g., 2024)
- m: Two-digit month (e.g., 01 for January)
- d: Two-digit day (e.g., 29)
- H: Two-digit hour in 24-hour format (e.g., 14)
- i: Two-digit minute (e.g., 30)
- s: Two-digit second (e.g., 00)
Creating Date Objects:
PHP’s DateTime class provides a more flexible way to work with dates and times:
<?php
$date = new DateTime('2024-01-29');
echo $date->format('Y-m-d'); // Output: 2024-01-29
?>
Manipulating Dates:
You can perform various operations on DateTime objects using methods like modify() and add():
<?php
$date->modify('+1 day');
echo $date->format('Y-m-d'); // Output: 2024-01-30
$date->add(new DateInterval('P1M')); // Add 1 month
echo $date->format('Y-m-d'); // Output: 2024-02-29
?>
Timezones:
When working with timezones, you can set the default timezone using date_default_timezone_set() or specify timezones explicitly:
<?php
date_default_timezone_set('America/New_York');
$date = new DateTime('now');
echo $date->format('Y-m-d H:i:s');
?>
Timestamps:
Timestamps represent the number of seconds since the Unix Epoch (January 1, 1970, 00:00:00 GMT). You can get the current timestamp using time():
<?php
$timestamp = time();
echo $timestamp;
?>
Parsing Dates:
To parse date strings into Unix timestamps, you can use strtotime():
<?php
$timestamp = strtotime('2024-01-29 14:30:00');
echo date('Y-m-d H:i:s', $timestamp);
?>
PHP’s date and time functions offer powerful capabilities for managing dates, times, and timezones in web applications, allowing developers to handle a wide range of scenarios related to date and time manipulation.
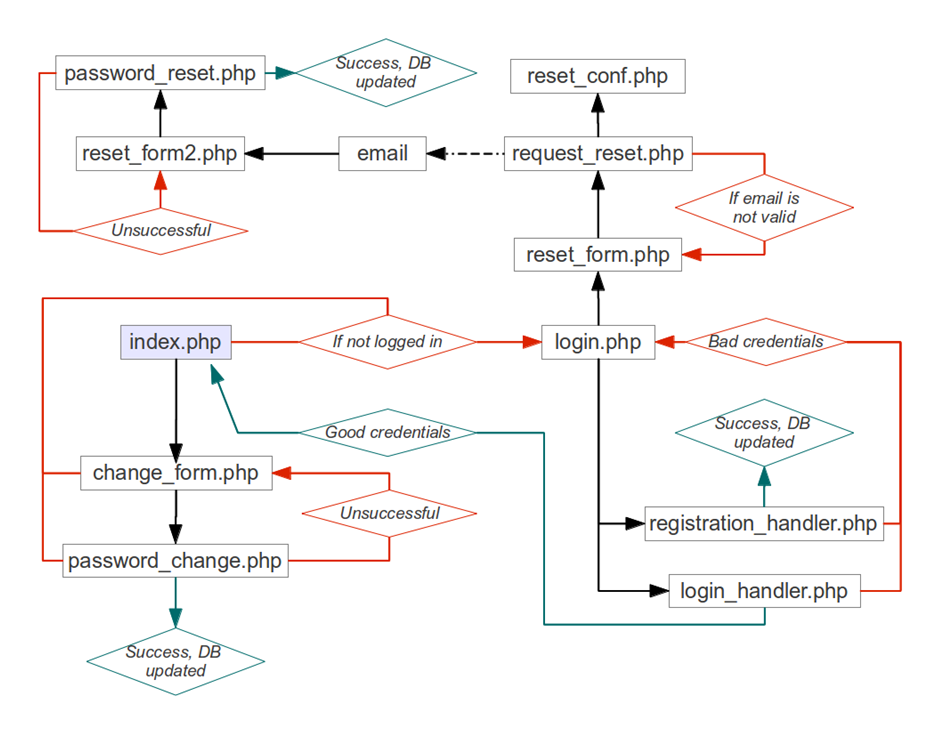