Colspan
Definition:
In HTML, the colspan attribute is used to specify how many columns a table cell should span horizontally within a table row. It allows you to merge multiple columns into a single cell. The colspan attribute is often used in conjunction with the <td> (table data) or <th> (table header) elements.
Syntax:
<table border="1">
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
</tr>
<tr>
<td>Row 1, Cell 1</td>
<td colspan="2">Row 1, Cell 2 and Cell 3 merged</td>
</tr>
<tr>
<td>Row 2, Cell 1</td>
<td>Row 2, Cell 2</td>
<td>Row 2, Cell 3</td>
</tr>
</table>
In this example, the second row contains a cell with colspan=”2″. This means that the cell spans two columns (Header 2 and Header 3) in that specific row, effectively merging them into a single cell. The resulting table will have the first row with three columns, the second row with two columns, and the third row with three columns.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>HTML table</h1>
<table border="1">
<tr>
<th>Employee ID</th>
<th>Name</th>
<th colspan="2">Contact</th>
</tr>
<tr>
<td>001</td>
<td>John Doe</td>
<td>89345-12345</td>
<td>89745-11145</td>
</tr>
<tr>
<td>002</td>
<td>Jane Smith</td>
<td>89745-15545</td>
<td>90345-12345</td>
</tr>
</table>
</body>
Output:
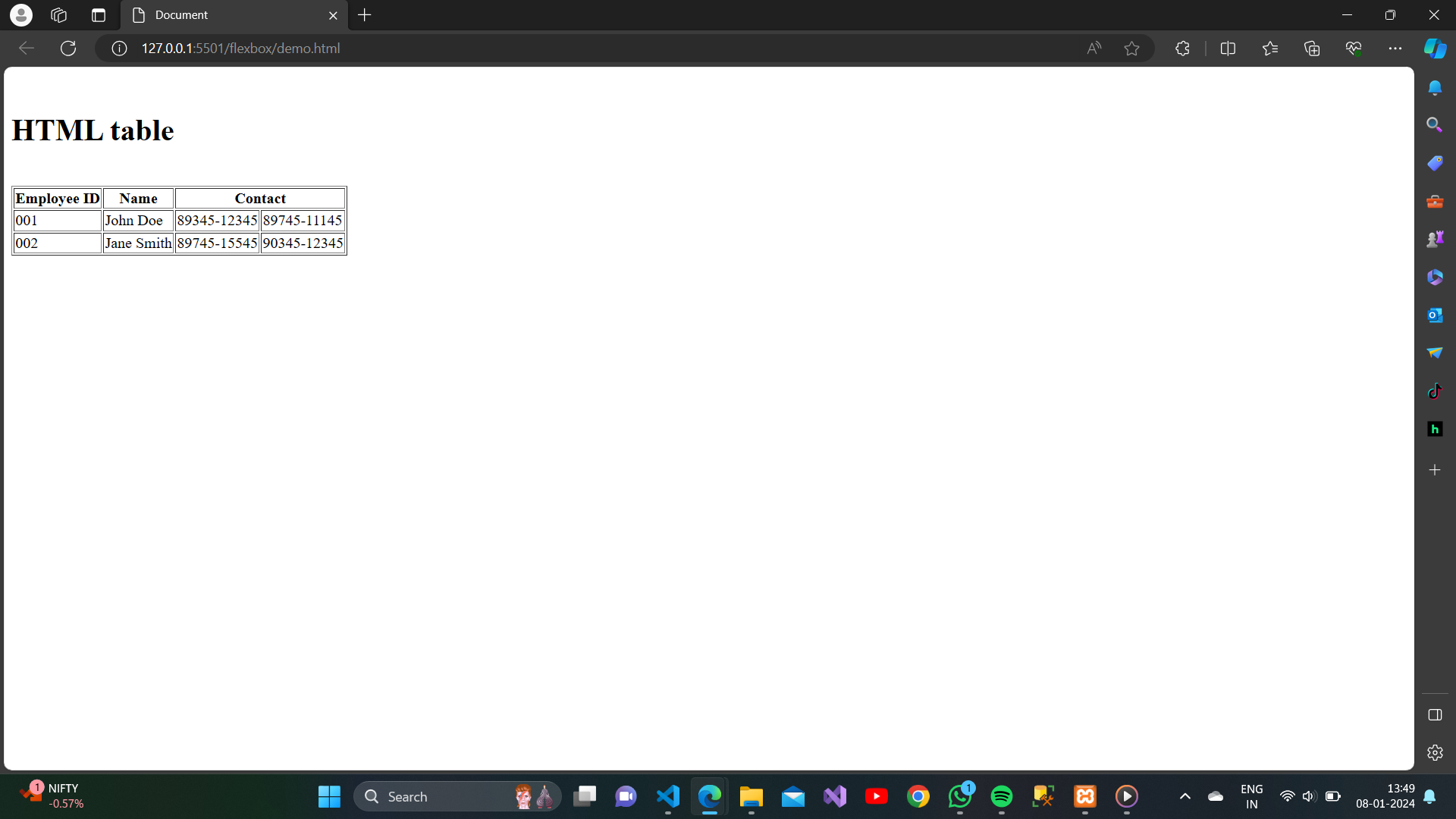
Rowspan
Definition:
In HTML, the rowspan
attribute is used to specify how many rows a table cell should span vertically within a table column. It allows you to merge multiple rows into a single cell. The rowspan
attribute is often used in conjunction with the <td>
(table data) or <th>
(table header) elements.
Syntax:
<table border="1">
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
</tr>
<tr>
<td rowspan="2">Row 1, Cell 1 and Cell 2 merged</td>
<td>Row 1, Cell 3</td>
<td>Row 1, Cell 4</td>
</tr>
<tr>
<td>Row 2, Cell 3</td>
<td>Row 2, Cell 4</td>
</tr>
</table>
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>HTML table</h1>
<table border="1">
<tr>
<th>Employee ID</th>
<th>Name</th>
<th>Contact</th>
<th>Department</th>
</tr>
<tr>
<td>001</td>
<td>John Doe</td>
<td>123-456-7890</td>
<td rowspan="2">Computer Science</td>
</tr>
<tr>
<td>002</td>
<td>Jane Smith</td>
<td>987-654-3210</td>
</tr>
</table>
</body>
</html>
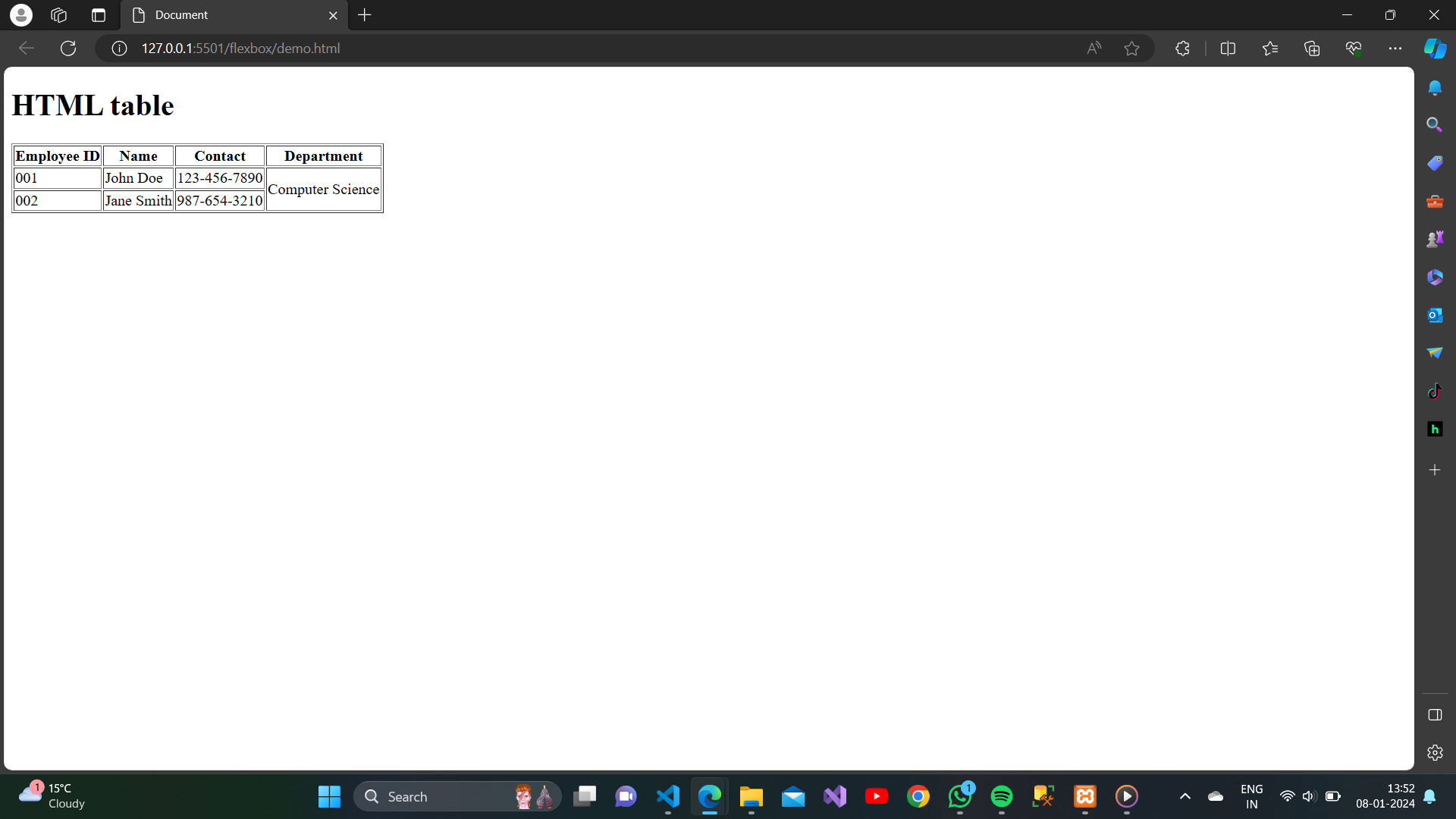