About Lesson
Attributes of Input:
Input elements can have various attributes to customize their behavior.
- name: Specifies the name of the input element for form submission.
- type: Defines the type of input control. Common values include “text,” “password,” “checkbox,” “radio,” etc.
- for: used in label to link the label with the input or textarea.
- id: used to identify a particular element.
- placeholder: Provides a hint or example text for the input field.
- required: Makes the field mandatory for form submission.
- disabled: Disables the input element and the value is not submitted at all.
- readonly: Prevents user input but allows form submission.
- value: Sets the default or initial value of the input.
- maxlength: Limits the maximum number of characters for text inputs.
- minlength: Limits the minimum number of characters for text inputs.
- min and max: Sets the range for numeric inputs.
- step: Specifies the increment for numeric inputs.
- checked: Pre-selects checkboxes or radio buttons.
- autofocus: Automatically focuses on the input when the page loads.
- autocomplete: Controls whether browser auto-completion is enabled.
- pattern: Defines a regular expression pattern for input validation.
- multiple: Allows multiple file selection in file inputs.
Example:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Attributes Example</title>
</head>
<body>
<form action="/submit" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" placeholder="Enter your username" required autofocus
autocomplete="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" placeholder="Enter your password" required
autocomplete="current-password">
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required autocomplete="email">
<br>
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="18" max="100" step="1" required>
<br>
<label for="file">Select multiple files:</label>
<input type="file" id="file" name="file" multiple>
<br>
<label for="bio">Biography:</label>
<br>
<textarea id="bio" name="bio" rows="4" cols="50" placeholder="Write something about yourself"></textarea>
<br>
<input type="checkbox" id="subscribe" name="subscribe" checked>
<label for="subscribe">Subscribe to newsletter</label>
<br>
<input type="radio" id="male" name="gender" value="male" checked>
<label for="male">Male</label>
<br>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label>
<br>
<button type="submit">Submit</button>
</form>
</body>
</html>
Example:
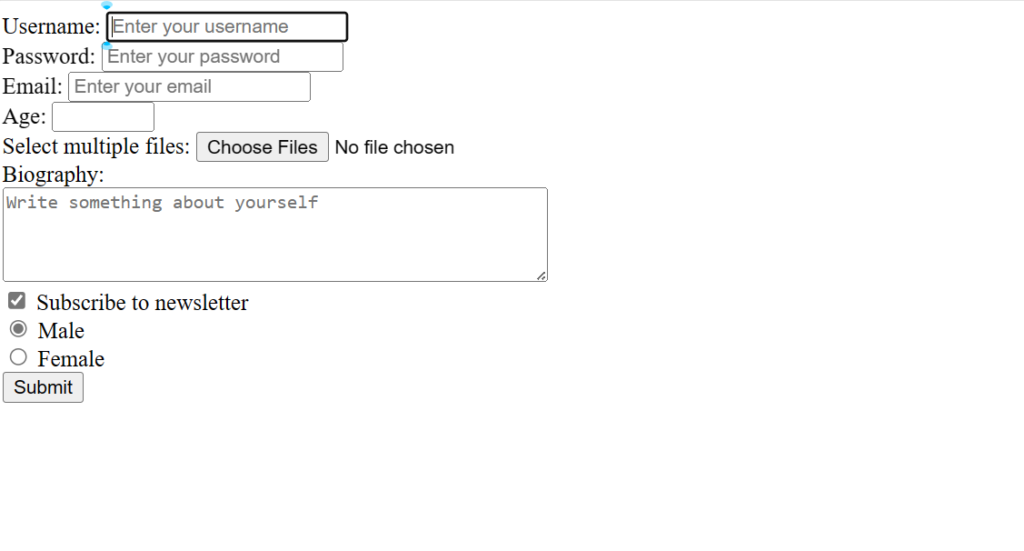