About Lesson
Definition:
In web development, an HTML table is an element used to display data in a tabular format of rows and columns on a web page. It allows you to organize and present information in a structured way, making it easier for users to read and comprehend the data. Tables are created using HTML tags and can include headers, data cells, and various formatting elements.
Syntax:
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Row 1, Cell 1</td>
<td>Row 1, Cell 2</td>
</tr>
<tr>
<td>Row 2, Cell 1</td>
<td>Row 2, Cell 2</td>
</tr>
</table>
In this Structure:
<table>
: Defines the start of the table.<tr>
: Defines a table row. It contains one or more<th>
(table header) or<td>
(table data) elements.<th>
: Represents a header cell in the table. Header cells are typically used for column or row labels.<td>
: Represents a data cell in the table. Data cells contain the actual content of the table.
You can have multiple rows (<tr>
) in a table, and within each row, you can have multiple header cells (<th>
) and data cells (<td>
).
Example:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>HTML table</h1>
<h2>Here's an example of an HTML table displaying information about students in a college. In this table, each row represents a student, and the columns represent different attributes such as student ID, name, age, and course:</h2>
<table border="1">
<tr>
<th>Student ID</th>
<th>Name</th>
<th>Age</th>
<th>Course</th>
</tr>
<tr>
<td>001</td>
<td>John Doe</td>
<td>20</td>
<td>Computer Science</td>
</tr>
<tr>
<td>002</td>
<td>Jane Smith</td>
<td>22</td>
<td>Engineering</td>
</tr>
<tr>
<td>003</td>
<td>Alice Johnson</td>
<td>21</td>
<td>Mathematics</td>
</tr>
<tr>
<td>004</td>
<td>Michael Brown</td>
<td>23</td>
<td>Physics</td>
</tr>
</table>
</body>
</html>
Output:
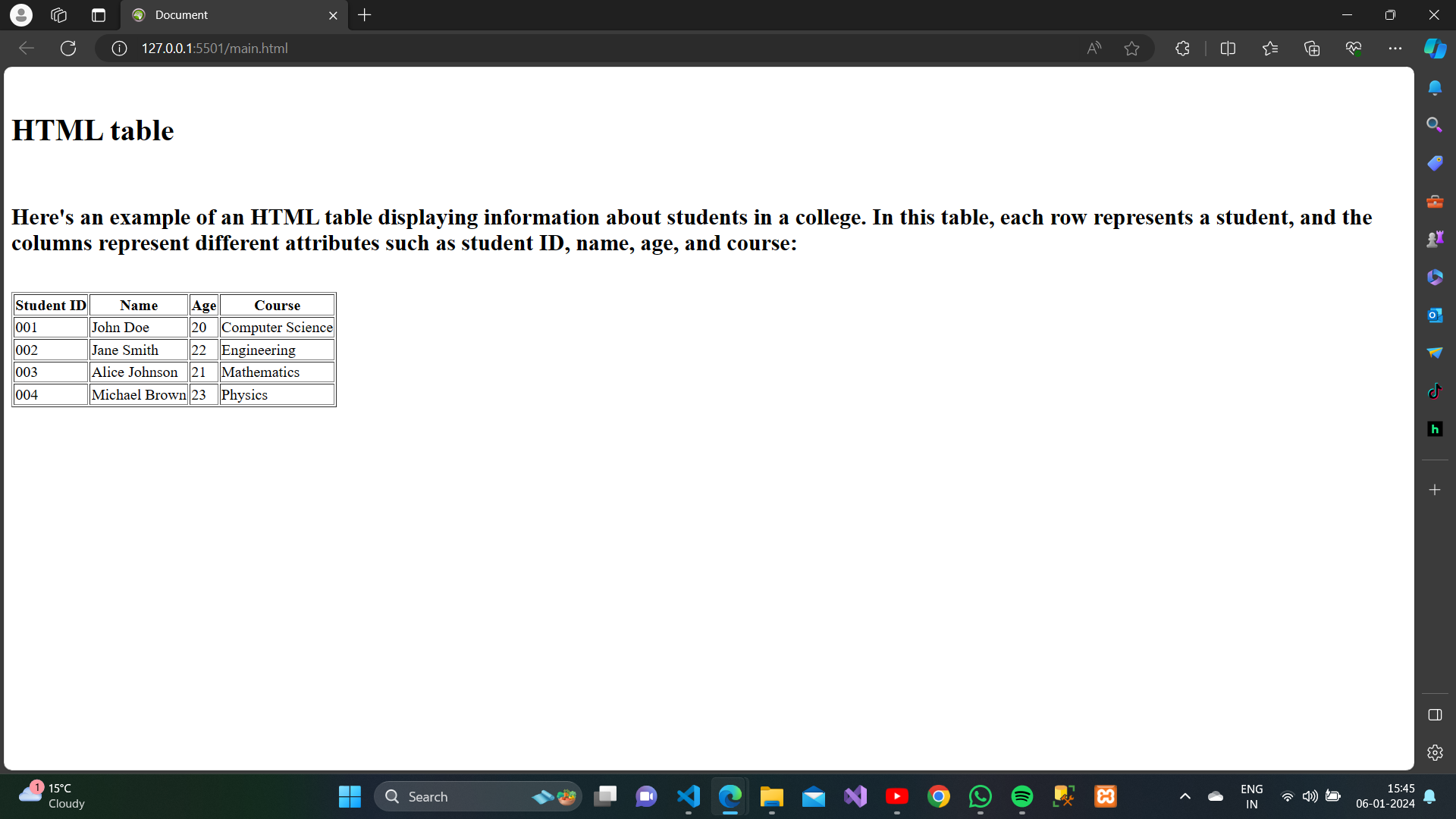